Factory Design Pattern In Java
Factory Design Pattern is one of the most commonly used design patterns. It falls under the category of Creational Design Patterns.
Overview
Analogy
As the name suggests, Factory Design Pattern is based on real time Factory concept. As we know that factory manufactures products as per the requirements. It does not expose the internal details of manufacturing. Also, in case of requirements for newer products, it manufactures them as well.
Similarly, Factory Design Pattern enables us to create objects using a factory class without exposing the creation logic to the client and thus providing abstraction with flexibility to extension.
When a client needs an object it asks the factory for it by providing some information on the type of object it requires. Factory method in factory class acts as a single place to create the objects from concrete classes based on the client’s input and return them as objects of interface or abstract types.
Virtual Constructor
This pattern replaces the direct object construction calls (using new operator) from client with calls to factory method. Since it replaces direct constructor calls, it is also known as Virtual Constructor.
GoF Design Patterns
According to GoF (Gangs of Four) Design Patterns,
Factory Design Pattern defines an interface for creating an object, but let subclasses decide which class to instantiate. The Factory method lets a class defer instantiation to subclasses.
When to use Factory Design Pattern?
- When we need to create objects of types which may be sub-classed.
- When client needs to create an object based on some input parameter and need not know how the object is created
- Also, a good choice when we don’t know the exact types before hand when starting on a project. This would provide ease to extensibility.
How to implement Factory Design Pattern?
- Create a super class (an interface)
- Create sub class(es) (requirement based):
- Concrete A
- Concrete B
- Concrete C
- Factory Class with factory method that accepts some input parameter (to decide the type of object) to return the required object as object of interface
- Create an Enum that would be used to define types based on which factory method would create an object of concrete class
- Client code(main class) which creates an object using factory method of factory class by providing some input parameter and then use it
Code Example
Now we know what are the classes required to build a Factory Design Pattern.
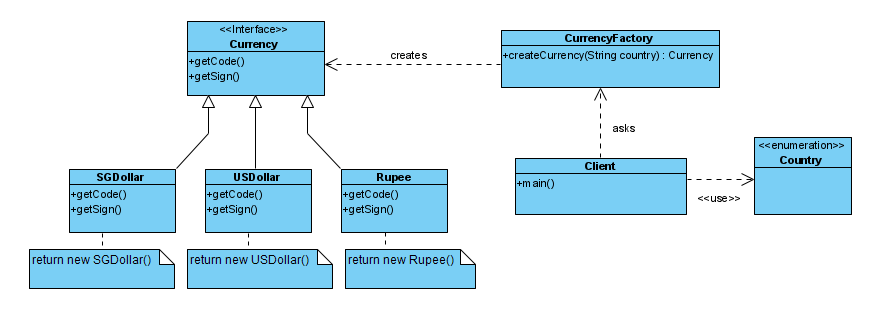
-
Let’s first create an interface named
Currency
public interface Currency { String getCode(); String getSign(); }
-
Create concrete classes
Rupee
,SGDollar
, andUSDollar
implementingCurrency
interfacepublic class Rupee implements Currency { @Override public String getCode() { return "INR"; } @Override public String getSign() { return "₹"; } } public class SGDollar implements Currency { @Override public String getCode() { return "SGD"; } @Override public String getSign() { return "S$"; } } public class USDollar implements Currency { @Override public String getCode() { return "USD"; } @Override public String getSign() { return "$"; } }
-
Create an Enum
Country
for input parameter to factory methodpublic enum Country { INDIA, SINGAPORE, USA, CANADA }
-
Finally create a factory class
CurrencyFactory
public class CurrencyFactory { public static Currency createCurrency(String country) { if(country.equalsIgnoreCase(Country.SINGAPORE.toString())) { return new SGDollar(); } else if(country.equalsIgnoreCase(Country.INDIA.toString()) { return new Rupee(); } else if(country.equalsIgnoreCase(Country.USA.toString())) { return new USDollar(); } throw new IllegalArgumentException(String.format("No currency found for '%s'", country)); } }
-
Let’s create a
Client
(Main Class) to test our Factorypublic class Client { public static void main(String [] args) { Currency indiaCurrency = CurrencyFactory.createCurrency(Country.INDIA.toString()); Currency singaporeCurrency = CurrencyFactory.createCurrency(Country.SINGAPORE.toString()); Currency usaCurrency = CurrencyFactory.createCurrency(Country.USA.toString()); //Currency canadaCurrency = CurrencyFactory.createCurrency(Country.CANADA.toString()); //Exception in thread "main" java.lang.IllegalArgumentException: No currency found for 'CANADA' System.out.println(indiaCurrency.getCode()); System.out.println(indiaCurrency.getSign()); System.out.println(singaporeCurrency.getCode()); System.out.println(singaporeCurrency.getSign()); System.out.println(usaCurrency.getCode()); System.out.println(usaCurrency.getSign()); } }
OutputINR ₹ SGD S$ USD $
Advantages of Factory Design Pattern
- Loose Coupling: Introduces loose coupling between classes. Hence, involves programming against abstract entities rather than concrete implementations.
- Encapsulation: Good approach to encapsulation. Factory method used to create different objects from factory encapsulates the object creation code.
- Abstraction: Provides abstraction between implementation and client classes through inheritance.
- Single Responsibility Principle: Object creation is done at one place and this separates out the object creation code from actual usage. Any changes in object creation in future would require change in only place.
- Open/Closed Principle: Further to extend the functionality of factory to support newer type would be a small change i.e. adding a new subclass and corresponding object creation logic in factory method.