Custom HTML Error Page using Thymeleaf
In this article, we’ll learn how to customize default Whitelabel Error Page in Spring Boot using Thymeleaf HTML templates
Whitelabel Error Page
Whitelabel error page is default error page generated by Spring Boot when no custom error page is provided.
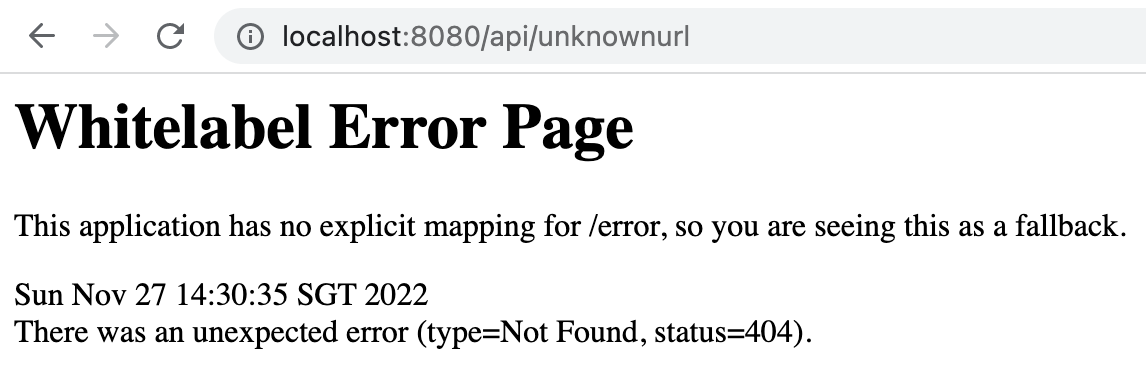
If you don’t like this default behavior and looking for custom HTML pages to be displayed for different type of HTTP errors then Thymeleaf error templates are simplest approach to follow.
Thymeleaf Error Templates
You create Thymeleaf HTML error template under resources/templates
directory:-
- Generic error template required with name
error.html
- Specific error templates are optional under
errors
directory with name<error_code>.html
for e.g.404.html
for Not found error,500.html
for Internal server error, etc.
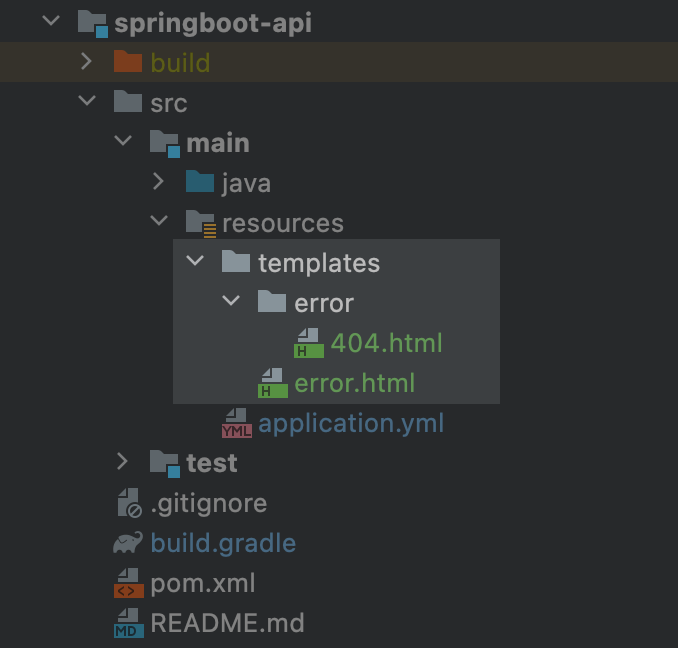
Generic Error Template
If specific error template not found then fallback to generic template for all types of HTTP error codes.
resources/templates/error.html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>Something went wrong!</title>
</head>
<body>
<h1>Generic Error - <span th:text="${status}">Status</span></h1>
<p>Sorry for the inconvenience. Please contact the administrator.</p>
<br/>
<ul>
<li>Timestamp: <span th:text="${timestamp}">Timestamp</span></li>
<li>Path: <span th:text="${path}">Path</span></li>
<li>Error: <span th:text="${error}">Error</span></li>
</ul>
</body>
</html>
Specific error template 500.html doesn’t exist, display error.html
Specific Error Template
If specific error template found for e.g. 404.html, display it for 404 error!
We imported bootstrap css in the 404 error page to look more elegant.
resources/templates/errors/404.html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1">
<!-- Bootstrap -->
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@3.4.1/dist/css/bootstrap.min.css" integrity="sha384-HSMxcRTRxnN+Bdg0JdbxYKrThecOKuH5zCYotlSAcp1+c8xmyTe9GYg1l9a69psu" crossorigin="anonymous">
<title>404 - resource not found</title>
</head>
<body>
<div class="container">
<h1><span class="label label-default">Resource Not Found Error - 404</span></h1>
<p>Requested resource was not found. Please contact the administrator.</p>
<table class="table table-bordered table-striped">
<tr>
<td>Timestamp</td>
<td th:text="${timestamp}">Timestamp</td>
</tr>
<tr>
<td>Path</td>
<td th:text="${path}">Path</td>
</tr>
<tr>
<td>Status</td>
<td th:text="${status}">Status</td>
</tr>
<tr>
<td>Error</td>
<td th:text="${error}">Error</td>
</tr>
<tr>
<td>Message</td>
<td th:text="${message}">Message</td>
</tr>
</table>
</div>
</body>
</html>
Specific error template 400.html exist, display it!
Thymeleaf Dependency
We need to add Thymeleaf dependency in the project for these error templates to work.
build.gradle
dependencies {
implementation 'org.springframework.boot:spring-boot-starter-thymeleaf'
}
pom.xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
Thats it! Once you add Thymeleaf dependency, it does all the magic.