Disable Whitelabel Error Page in Spring Boot
In this article, we’ll learn how to disable default Whitelabel Error Page in Spring Boot.
Whitelabel Error Page
Whitelabel error page appears when you hit a url which result into HTTP error. Whitelabel error page is generated by Spring Boot as a default mechanism when no custom error page is found.
Let’s hit a wrong or unknown url, which result into HTTP 404 error:-
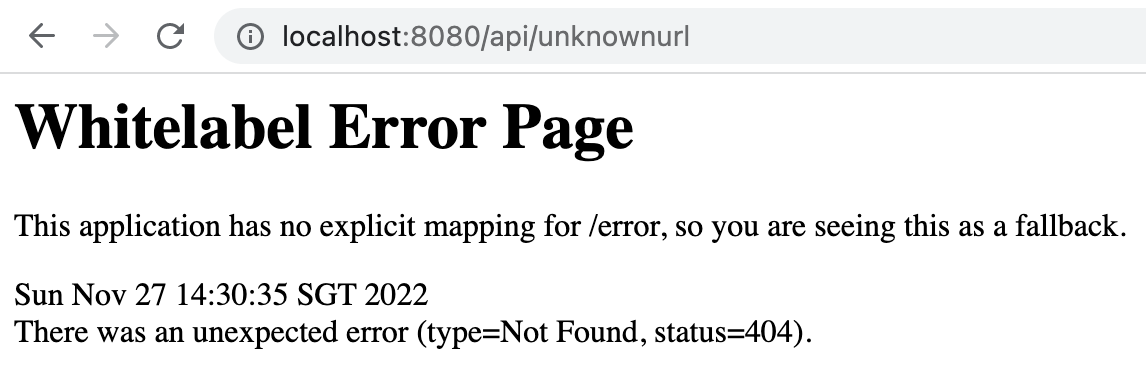
If you don’t want to see this default Whitelabel error page then it can be disabled using any one of these two properties:-
- Disable Whitelabel Error Server Property
server.error.whitelabel.enabled = false
- Exclude ErrorMvcAutoConfiguration
spring.autoconfigure.exclude = org.springframework.boot.autoconfigure.web.servlet.error.ErrorMvcAutoConfiguration ## For Spring Boot < 2.0 spring.autoconfigure.exclude = org.springframework.boot.autoconfigure.web.ErrorMvcAutoConfiguration
When Whitelabel error page is disabled and no custom error page is provided, you will see the error page generated by underlying web application server such as Tomcat, Undertow, Jetty etc.
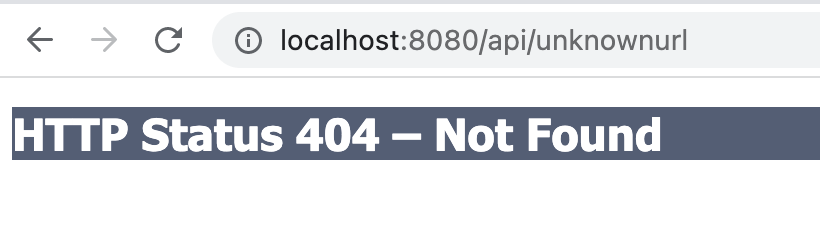
Follow the post for Customize Whitelabel HTML Error Page using Thymeleaf
Disable Whitelabel Error Server Property
You can disable the Whitelabel error page by setting the server.error.whitelabel.enabled
property to false
. This can be done from .properties file, .yml file, or through command-line parameters as follows:-
## application.properties
server.error.whitelabel.enabled = false
## application.yml
server:
error:
whitelabel:
enabled: false
$ java -jar -Dserver.error.whitelabel.enabled=false spring-boot-app-1.0.jar
$ java -jar spring-boot-app-1.0.jar --server.error.whitelabel.enabled=false
Exclude ErrorMvcAutoConfiguration
Another way to disable it via excluding the ErrorMvcAutoConfiguration
from auto configuration. This can be done easily from .properties file or .yml file
## application.properties
spring.autoconfigure.exclude = org.springframework.boot.autoconfigure.web.servlet.error.ErrorMvcAutoConfiguration
## application.yml
spring:
autoconfigure:
exclude: org.springframework.boot.autoconfigure.web.servlet.error.ErrorMvcAutoConfiguration
Alternatively, you can also use @EnableAutoConfiguration
annotation to exclude it.
import org.springframework.context.annotation.Configuration;
import org.springframework.boot.autoconfigure.web.servlet.error.ErrorMvcAutoConfiguration;
...
@Configuration
@EnableAutoConfiguration(exclude = {ErrorMvcAutoConfiguration.class})
public static MainApp { ... }
For Spring Boot < 2.0
Please note that for Spring Boot < 2.0, the ErrorMvcAutoConfiguration
class is located in package org.springframework.boot.autoconfigure.web
.
## application.properties
spring.autoconfigure.exclude = org.springframework.boot.autoconfigure.web.ErrorMvcAutoConfiguration
## application.yml
spring:
autoconfigure:
exclude: org.springframework.boot.autoconfigure.web.ErrorMvcAutoConfiguration